Given a binary tree root, a node X in the tree is named good if in the path from root to X there are
no nodes with a value greater than X.
Return the number of good nodes in the binary tree.
이진 트리가 주어졌을때 다음으로 탐색할 노드의 값이 현재 위치한 노드의 값보다 큰 숫자를 파악해서 리턴
※ Root 노드는 언제나 포함된다
https://leetcode.com/problems/count-good-nodes-in-binary-tree/description/
Example 1:
Input: root = [3,1,4,3,null,1,5]
Output: 4
Explanation: Nodes in blue are good.
Root Node (3) is always a good node.
Node 4 -> (3,4) is the maximum value in the path starting from the root.
Node 5 -> (3,4,5) is the maximum value in the path
Node 3 -> (3,1,3) is the maximum value in the path.
Example 2:
Input: root = [3,3,null,4,2]
Output: 3
Explanation: Node 2 -> (3, 3, 2) is not good, because "3" is higher than it.
Example 3:
Input: root = [1]
Output: 1
Explanation: Root is considered as good.
DFS를 통해 탐색 도중 현재 최대값을 보내 더 높다면 count하고 아니면 다음 노드로 이동하는 문제
1. ans 저장
2. 현재 최대값이랑 확인하는 Node 값 확인해서 최댓값 최신화
3. 자식 노드 탐색
class Solution:
def goodNodes(self, root: TreeNode) -> int:
ans = 0
def dfs(root, maxNum):
if not root: return
if root.val >= maxNum:
nonlocal ans
ans += 1
maxNum = root.val
dfs(root.left, maxNum)
dfs(root.right, maxNum)
dfs(root, root.val)
return ans
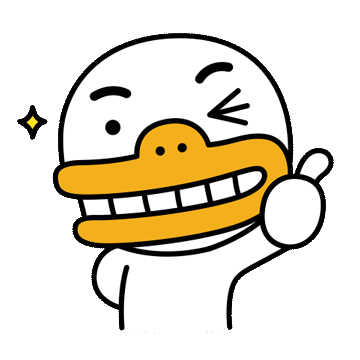
반응형
'알고리즘 & 자료구조 > Leetcode' 카테고리의 다른 글
[Leetcode 230] - Kth Smallest Element in a BST w/ Python (0) | 2024.04.10 |
---|---|
[Leetcode 98] - Validate Binary Search w/ Python (0) | 2024.04.10 |
[Leetcode 199] - Binary Tree Right Side View w/ Python (1) | 2024.04.10 |
[Leetcode 102] - Binary Tree Level Order Traversal w/ Python (0) | 2024.04.09 |
[Leetcode 235] - Lowest Common Ancestor of a Binary Search Tree w/ Python (0) | 2024.04.09 |